ImGuiUI is an extention to the popular Dear Imgui library of Omar Ocornut to make it easier to create desktop applications with the docking branch. It can be used in a wide variety of applications like level editors, profilers, raytracers or even spotify clones!
First of all, a huge thanks to Omar for creating and maintaining Dear Imgui. It’s an amazing library!
Than, what is ImGuiUI? ImGuiUI is a small piece of helper software that makes it easier to create neat looking applications. It is easy to set up and use, and leaves you free to do whatever you want within your application.
Github over here.
Some examples:
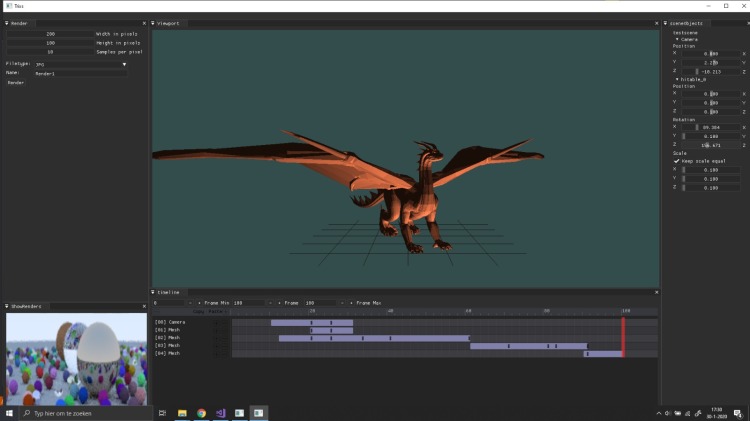

How does it work?
ImGuiUI comes with 2 main classes, the windowManager and the UIwindow. The windowManager keeps track of all the windows and updates them. UIwindow is a pure virtual class that functions as a template for your windows. Override the update function and add all the standard ImGui there.
Example
In the example directory an example is given to use the extension with GLFW. It can be easily converted for SDL and others.
The example consists of 3 classes. The first one is the GLFW implementation of the windowmanager. In order to use GLFW with ImGui there are some extra functions we need to call on startup and render. That’s why we inherit from the base class and create our own GLFW window manager:
class GLFWWindowManager : public ImGuiUI::WindowManager
{
public:
GLFWWindowManager();
~GLFWWindowManager();
virtual void Update();
bool shouldClose() { return mainWindow.WindowShouldClose(); }
private:
Window mainWindow;
};
As you can see, the GLFWWindowManager has a private window object. It’s just a GLFWwindow wrapper class, I don’t think it needs an explanation but if it does let me know. The constructor of our new class handles the setup of the imgui glfw implementation, which is shut down in the deconstructor:
GLFWWindowManager::GLFWWindowManager() : mainWindow{ SCR_WIDTH, SCR_HEIGHT }
{
ImGui_ImplGlfw_InitForOpenGL(mainWindow.getRawWindow(), true);
const char* glsl_version = "#version 410";
ImGui_ImplOpenGL3_Init(glsl_version);
}
GLFWWindowManager::~GLFWWindowManager()
{
ImGui_ImplOpenGL3_Shutdown();
}
Than there is the update function. In this function we extend the main update function to add all the GLFW implementation calls. First we create a new frame, then we update the base class, and to finish it off we render.
void GLFWWindowManager::Update()
{
ImGui_ImplOpenGL3_NewFrame();
ImGui_ImplGlfw_NewFrame();
ImGuiUI::WindowManager::Update();
ImGuiIO& io = ImGui::GetIO(); (void)io;
if (io.ConfigFlags & ImGuiConfigFlags_ViewportsEnable)
{
GLFWwindow* backup_current_context = glfwGetCurrentContext();
ImGui::UpdatePlatformWindows();
ImGui::RenderPlatformWindowsDefault();
glfwMakeContextCurrent(backup_current_context);
}
ImGui_ImplOpenGL3_RenderDrawData(ImGui::GetDrawData());
mainWindow.Draw();
}
Now this is all nice, but we need something to render. That’s where this example window is for:
class exampleWindow : public ImGuiUI::UIWindow
{
public:
exampleWindow()
{
count = 0;
}
~exampleWindow() {}
void update() override
{
if (showing)
{
if (!ImGui::Begin("Settings", &showing))
{
ImGui::End();
}
else
{
ImGui::Text("This is an example window");
ImGui::Text("Dock this window!");
ImGui::Separator();
ImGui::Text("You can create your own windows for any purpose");
if (ImGui::Button("This is a button"))
{
count++;
}
ImGui::Text("Count: %d", count);
ImGui::End();
}
}
}
private:
int count;
};
This class inherits from the base UIWindow and implements the update function with some ImGui ui elements.
In the source file we can see how these classes are used to setup an application:
#include "GLFWExample.h"
int main()
{
GLFWWindowManager ui;
ui.AddWindow(std::unique_ptr<exampleWindow>(new exampleWindow()));
while (!ui.shouldClose())
{
ui.Update();
}
return 0;
}
Help out
This code is far from perfect. If you found a bug, created an improvement or have a suggestion please feel free to create an issue or pull request. This project is only a small addition to the huge Dear Imgui Library, please consider helping out on it’s development here.
See you,
Ruurd